아래 소스코드를 통해 학습을 하면, 정확도는 50%도 안 나온다.
이유
CIFAR-10 dataset은 컬러 이미지 데이터 -> 이미지를 1차원으로 flatten 시킴으로써 이미지의 지역적인 특징을 학습하기가 어렵고, 3개 채널의 컬러 이미지가 1차원으로 펼쳐짐으로 인해서 상실되는 정보가 많기 때문
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
|
import torch
import numpy as np
import os
import matplotlib.pyplot as plt
import torch.nn as nn
import torch.nn.functional as F
from torchvision import transforms, datasets
import torch.nn.init as init
os.environ['KMP_DUPLICATE_LIB_OK'] = 'True'
BATCH_SIZE = 64
EPOCHS = 10
if torch.cuda.is_available():
DEVICE = torch.device('cuda')
else:
DEVICE = torch.device('cpu')
print(DEVICE)
train_dataset = datasets.CIFAR10(root="./data/CIFAR_10",
train=True,
download=True,
transform=transforms.ToTensor())
test_dataset = datasets.CIFAR10(root="./data/CIFAR_10",
train=False,
download=True,
transform=transforms.ToTensor())
train_loader = torch.utils.data.DataLoader(dataset=train_dataset,
batch_size=BATCH_SIZE,
shuffle=True)
test_loader = torch.utils.data.DataLoader(dataset=test_dataset,
batch_size=BATCH_SIZE,
shuffle=False)
# 다운로드 받은 데이터셋 확인
for (x_train, y_train) in train_loader:
print('x_train: ', x_train.size(), ' data_type: ', x_train.type())
print('y_train: ', y_train.size(), ' data_type: ', y_train.type())
break
fig = plt.figure(figsize=(5, 1))
for i in range(5):
plt.subplot(1, 5, i + 1)
plt.axis('off')
plt.imshow(np.transpose(x_train[i], (1, 2, 0)))
plt.title("class: " + str(y_train[i].item()))
plt.show()
class MLP(nn.Module):
def __init__(self):
super(MLP, self).__init__()
self.dense1 = nn.Linear(32*32*3, 512)
self.dense2 = nn.Linear(512, 256)
self.dense3 = nn.Linear(256, 10)
def forward(self, x):
x = x.view(-1, 32*32*3)
x = self.dense1(x)
x = F.relu(x)
x = nn.BatchNorm1d(512)(x)
x = self.dense2(x)
x = F.relu(x)
x = nn.BatchNorm1d(256)(x)
x = self.dense3(x)
x = F.softmax(x, dim=1)
return x
def weight_initializer(m):
if isinstance(m, nn.Linear):
init.kaiming_uniform_(m.weight.data)
model = MLP().to(DEVICE)
model.apply(weight_initializer) #가중치 초기화 기법을 사용
optimizer = torch.optim.Adam(model.parameters(), lr=1e-3)
criterion = nn.CrossEntropyLoss()
print(model)
def train(model, train_loader, optimizer, interval):
model.train()
for idx, (image, label) in enumerate(train_loader):
image = image.to(DEVICE)
label = label.to(DEVICE)
optimizer.zero_grad()
output = model(image)
loss = criterion(output, label)
loss.backward()
optimizer.step()
if idx % interval == 0:
print('train epoch: {}, {}/{} train_loss: {}'
.format(epoch, idx*len(image), len(train_loader.dataset), loss.item()))
def evaluate(model, test_loader):
model.eval()
test_loss = 0
right = 0
with torch.no_grad():
for image, label in test_loader:
image = image.to(DEVICE)
label = label.to(DEVICE)
output = model(image)
test_loss += criterion(output, label).item()
pred = output.max(1, keepdim=True)[1]
right += pred.eq(label.view_as(pred)).sum().item()
test_loss /= len(test_loader.dataset)
test_acc = right/len(test_loader.dataset) * 100
return test_loss, test_acc
for epoch in range(1, EPOCHS+1):
train(model, train_loader, optimizer, 200)
test_loss, test_acc = evaluate(model, test_loader)
print("test_loss: {}, test_acc: {}".format(test_loss, test_acc))
|
cs |
위의 소스 코드를 실행시키면, 아래와 같은 결과값을 얻을 수 있다.
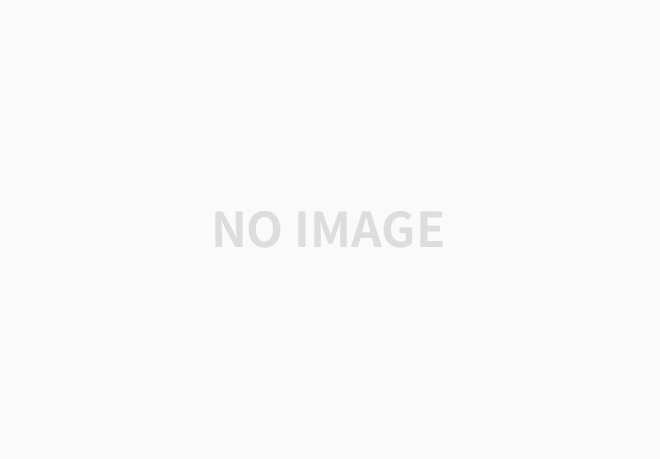
반응형
'머신러닝_딥러닝 > Pytorch' 카테고리의 다른 글
CNN 2탄 (CIFAR-10 dataset) (0) | 2021.10.17 |
---|---|
CNN 1탄 (CIFAR-10 dataset) (0) | 2021.10.17 |
오토인코더(AutoEncoder) 구현 기초예제 (0) | 2021.10.17 |
오토인코더 (AutoEncoder) (0) | 2021.10.17 |
MLP모델 설계 및 학습 2탄 (MNIST dataset) (0) | 2021.10.16 |