1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
|
import numpy as np
# 로지스틱 회귀 (Logistic regression)
# 이진 분류 알고리즘, 지도 학습 알고리즘
# 회귀의 출력값을 sigmoid 함수의 입력값으로 사용하여 0~1사이의 값을 얻은 다음,
# 그 값이 0.5 이상이면 출력값 y를 1로 정의하고,
# 0.5 미만이면 y를 0으로 정의하여, 분류시스템을 구현
# 손실함수 : binary cross-entropy
## 로지스틱 회귀 기초 예제 ##
# 학습 데이터
x_train = np.array([1, 3, 5, 6, 7, 8, 10, 12, 14, 17]).reshape(10, 1)
y_train = np.array([0, 0, 0, 0, 0, 1, 1, 1, 1, 1]).reshape(10, 1)
# 가중치와 바이어스 초기화
W = np.random.rand(1, 1)
b = np.random.rand(1)
print("W = ", W, ", b = ", b)
# 시그모이드 함수 & 손실함수
def sigmoid(x):
return 1/(1+np.exp(-x))
def loss_func(x, t):
delta = 1e-6 # log가 무한대로 발산하는 것을 막아준다
z = np.dot(x, W) + b
y = sigmoid(z)
return -np.sum(t*np.log(y+delta) + (1-t)*np.log((1-y)+delta))
# 수치미분 정의
def numerical_derivative(func, x):
delta = 1e-4
grad = np.zeros_like(x)
it = np.nditer(x, flags=['multi_index'], op_flags=['readwrite'])
while not it.finished:
idx = it.multi_index
temp = x[idx]
x[idx] = float(temp) + delta
f1 = func(x)
x[idx] = float(temp) - delta
f2 = func(x)
grad[idx] = (f1 - f2) / (2 * delta)
x[idx] = temp
it.iternext()
return grad
# Utility 함수
def error_eval(x, t):
delta = 1e-6 # log가 무한대로 발산하는 것을 막아준다
z = np.dot(x, W) + b
y = sigmoid(z)
return -np.sum(t * np.log(y + delta) + (1 - t) * np.log((1 - y) + delta))
def predict(x):
z = np.dot(x, W) + b
y = sigmoid(z)
if y >= 0.5:
result = 1
else:
result = 0
return result
# 학습하기
learning_rate = 1e-2
f = lambda x: loss_func(x_train, y_train)
for step in range(10001):
W -= learning_rate * numerical_derivative(f, W)
b -= learning_rate * numerical_derivative(f, b)
if (step % 500) == 0:
print("step = ", step, ", error = ", error_eval(x_train, y_train), ", W = ", W, ", b =", b)
print(predict(4)) # 0
print(predict(13)) # 1
|
cs |
위 소스코드를 실행시키면 아래와 같은 출력값을 얻는다.
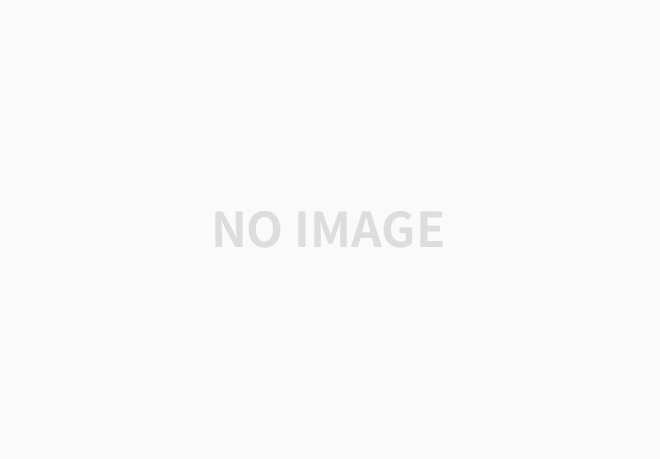
반응형
'머신러닝_딥러닝 > Tensorflow + Keras' 카테고리의 다른 글
XOR 문제 (딥러닝으로 해결) (0) | 2021.10.23 |
---|---|
XOR 문제 (0) | 2021.10.23 |
선형회귀 (Linear Regression) (0) | 2021.10.23 |
필수품 for Machine learning (0) | 2021.10.22 |
Tensorflow & Keras (0) | 2021.09.14 |